Plots With Python
Today we’ll learn about plotting 3D-graphs in Python using matplotlib. Matplotlib is an amazing module which not only helps us visualize data in 2 dimensions but also in 3 dimensions. 3D graphs represent 2D inputs and 1D output. The submodule we’ll be using for plotting 3D-graphs in python is mplot3d which is already installed when you install matplotlib. So, you need to make sure you have installed matplotlib to implement this tutorial.
Matplotlib is the most widely used scientific plotting library in Python. Commonly use a sub-library called matplotlib.pyplot.; The Jupyter Notebook will render plots inline if we ask it to using a “magic” command.% matplotlib inline import matplotlib.pyplot as plt. Create different types of plots (Scatter, Histograms, etc.) Change the esthetics of your figures (color, marker). Plot statistical distributions (such as Gaussian). Plot candlesticks charts. Read also: Introduction to Finance and Technical Indicators with Python. Please check the full notebook here. Happy Coding ♥ View Full Code.
So, Let’s get started!
Imports:
In this tutorial, we will be using the 3D plots in matplotlib. There are also other options like pandas3D. Feel free to play around with that too.
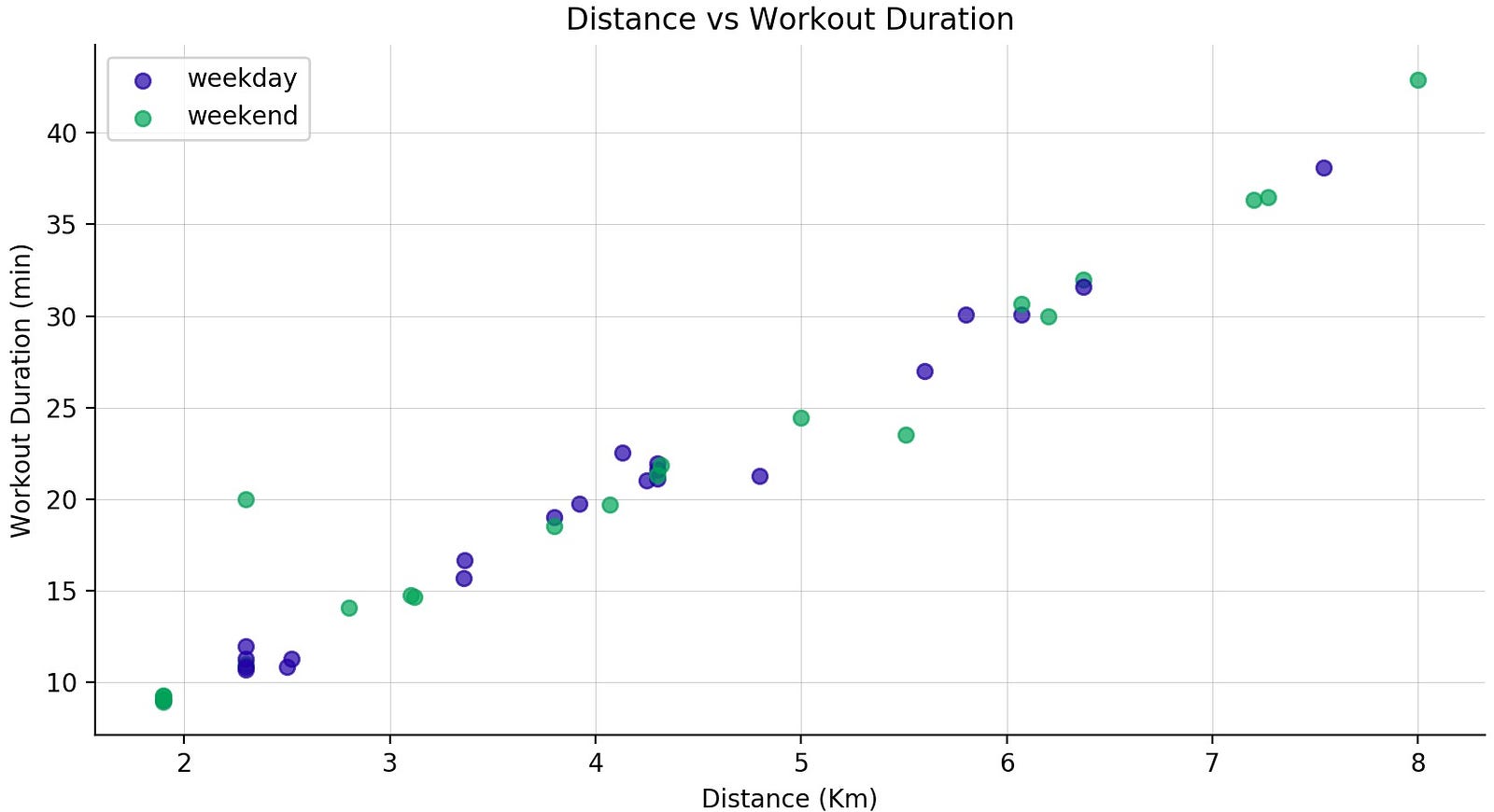
The submodule of matplotlib called mpl_toolkits is used to plot our 3D graphs. Check out its documentation here. We will also import matplotlib.pyplot itself too.
Making our dataset for 3d graph plotting
Now we need to get our x, y, and z values so that we can plot them. You can also make use of a csv or excel dataset to make it easier to visualize. Here, we’ll create three numpy arrays representing x, y, and z values.
For this, first import numpy and randint() function to create random datavalues:
We then define our numpy arrays by using the randint() function and list comprehension.
Now, let’s see what our values are :
Output:
Plotting our 3d graph in Python with matplotlib
Let’s first start by defining our figure
Now, to create a blank 3D axes, you just need to add “projection=’3d’ ” to plt.axes()
The output will look something like this:
Scatter Plots With Python
Now we add label names to each axis. To keep it simple, we’re just naming them ‘x’, ‘y’and ‘z’ respectively. Also, note that the function is ‘set_xlabel’ unlike in 2D plot where it is just ‘xlabel ‘
Finally, we get to the part where we plot the graph. The function used is plot3D().
Output:
Python Plot A List
We plotted the line graph here. There are a number of plotting techniques we can use like contour3D, scatter3D, plot_wireframe, and plot_surface, etc.
Also read:
How do I plot in 3D
a polyhedron using 3d vectors as vertices and arrays of vectors as faces